-
Notifications
You must be signed in to change notification settings - Fork 4
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
feature/AdSec_API_factorised_sample #9
Draft
PeterDebneyOasys
wants to merge
2
commits into
main
Choose a base branch
from
feature/AdSec_API_factorised_sample
base: main
Could not load branches
Branch not found: {{ refName }}
Loading
Could not load tags
Nothing to show
Loading
Are you sure you want to change the base?
Some commits from the old base branch may be removed from the timeline,
and old review comments may become outdated.
Draft
Changes from 1 commit
Commits
Show all changes
2 commits
Select commit
Hold shift + click to select a range
File filter
Filter by extension
Conversations
Failed to load comments.
Loading
Jump to
Jump to file
Failed to load files.
Loading
Diff view
Diff view
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,235 @@ | ||
# documentation: | ||
# https://arup-group.github.io/oasys-combined/adsec-api/index.html | ||
|
||
# Load the AdSec API | ||
import oasys.adsec | ||
|
||
# Import modules | ||
from Oasys.AdSec import ISection, IAdSec, ILoad, IVersion | ||
from Oasys.AdSec.DesignCode import CSA, IS456, EN1992 | ||
from Oasys.AdSec.IO.Graphics.Section import SectionImageBuilder | ||
from Oasys.AdSec.Reinforcement import IBarBundle, ICover | ||
from Oasys.AdSec.Reinforcement.Groups import ITemplateGroup, ILinkGroup, \ | ||
ISingleBars, IPerimeterGroup | ||
from Oasys.AdSec.Reinforcement.Layers import ILayerByBarCount, ILayerByBarPitch | ||
from Oasys.AdSec.StandardMaterials import Concrete, Reinforcement | ||
from Oasys.Profiles import IRectangleProfile, ICircleProfile, \ | ||
IPerimeterProfile, IPoint | ||
from Oasys.Units import Moment, MomentUnit | ||
from UnitsNet import Length, Force | ||
from UnitsNet.Units import LengthUnit, ForceUnit | ||
from reportlab.graphics import renderPM | ||
from svglib.svglib import svg2rlg | ||
|
||
# initialise default design code settings; override with code functions | ||
design_code = EN1992.Part1_1.Edition_2004.NationalAnnex.NoNationalAnnex | ||
material = Concrete.EN1992.Part1_1.Edition_2004. \ | ||
NationalAnnex.NoNationalAnnex.C32_40 | ||
bar_material = Reinforcement.Steel.EN1992.Part1_1.Edition_2004. \ | ||
NationalAnnex.NoNationalAnnex.S500B | ||
|
||
# initialised global variables | ||
section = ISection | ||
profile = 0 | ||
uls_deformation = 0 | ||
sls_deformation = 0 | ||
|
||
|
||
def code_ec2_gb(): | ||
global material | ||
global bar_material | ||
global design_code | ||
design_code = EN1992.Part1_1.Edition_2004.NationalAnnex.GB.Edition_2014 | ||
material = Concrete.EN1992.Part1_1.Edition_2004. \ | ||
NationalAnnex.GB.Edition_2014.C32_40 | ||
bar_material = Reinforcement.Steel.EN1992.Part1_1.Edition_2004. \ | ||
NationalAnnex.GB.Edition_2014.S500B | ||
|
||
|
||
def code_is456(): | ||
global material | ||
global bar_material | ||
global design_code | ||
design_code = IS456.Edition_2000 | ||
material = Concrete.IS456.Edition_2000.M25 | ||
bar_material = Reinforcement.Steel.IS456.Edition_2000.S500 | ||
|
||
|
||
def code_csa_s6(): | ||
global material | ||
global bar_material | ||
global design_code | ||
design_code = CSA.S6.Edition_2014 | ||
material = Concrete.CSA.S6.Edition_2014.C35 | ||
bar_material = Reinforcement.Steel.CSA.S6.Edition_2014.G30_18_400R | ||
|
||
|
||
def new_rectangle_section(depth, width, cover=30): | ||
global profile | ||
global material | ||
global section | ||
section_depth = Length(float(depth), LengthUnit.Millimeter) | ||
section_width = Length(float(width), LengthUnit.Millimeter) | ||
profile = IRectangleProfile.Create(section_depth, section_width) | ||
section = ISection.Create(profile, material) | ||
section.Cover = ICover.Create(Length(float(cover), LengthUnit.Millimeter)) | ||
|
||
|
||
def new_circle_section(diameter, cover=30): | ||
global profile | ||
global material | ||
global section | ||
section_diameter = Length(float(diameter), LengthUnit.Millimeter) | ||
profile = ICircleProfile.Create(section_diameter) | ||
section = ISection.Create(profile, material) | ||
section.Cover = ICover.Create(Length(float(cover), LengthUnit.Millimeter)) | ||
|
||
|
||
def add_links(diameter): | ||
global bar_material | ||
bar_dia = Length(float(diameter), LengthUnit.Millimeter) | ||
rebar = ILinkGroup.Create(IBarBundle.Create(bar_material, bar_dia)) | ||
section.ReinforcementGroups.Add(rebar) | ||
|
||
|
||
def add_perimeter_bars(diameter, number): | ||
global bar_material | ||
bar_dia = Length(float(diameter), LengthUnit.Millimeter) | ||
rebar = IPerimeterGroup.Create() | ||
bar_bundle = IBarBundle.Create(bar_material, bar_dia) | ||
layer = ILayerByBarCount.Create(number, bar_bundle) | ||
rebar.Layers.Add(layer) | ||
section.ReinforcementGroups.Add(rebar) | ||
|
||
|
||
def add_top_bars(diameter, number): | ||
global bar_material | ||
bar_dia = Length(float(diameter), LengthUnit.Millimeter) | ||
rebar = ITemplateGroup.Create(ITemplateGroup.Face.Top) | ||
bar_bundle = IBarBundle.Create(bar_material, bar_dia) | ||
layer = ILayerByBarCount.Create(number, bar_bundle) | ||
rebar.Layers.Add(layer) | ||
section.ReinforcementGroups.Add(rebar) | ||
|
||
|
||
def add_bottom_bars(diameter, number): | ||
global bar_material | ||
bar_dia = Length(float(diameter), LengthUnit.Millimeter) | ||
rebar = ITemplateGroup.Create(ITemplateGroup.Face.Bottom) | ||
bar_bundle = IBarBundle.Create(bar_material, bar_dia) | ||
layer = ILayerByBarCount.Create(number, bar_bundle) | ||
rebar.Layers.Add(layer) | ||
section.ReinforcementGroups.Add(rebar) | ||
|
||
|
||
def add_side_bars(diameter, spacing): | ||
global bar_material | ||
bar_dia = Length(float(diameter), LengthUnit.Millimeter) | ||
bar_spacing = Length(float(spacing), LengthUnit.Millimeter) | ||
rebar = ITemplateGroup.Create(ITemplateGroup.Face.Sides) | ||
bar_bundle = IBarBundle.Create(bar_material, bar_dia) | ||
layer = ILayerByBarPitch.Create(bar_bundle, bar_spacing) | ||
rebar.Layers.Add(layer) | ||
section.ReinforcementGroups.Add(rebar) | ||
|
||
|
||
def section_image(): | ||
global design_code | ||
global section | ||
flat_section = IAdSec.Create(design_code).Flatten(section) | ||
with open("section.svg", "w") as file: | ||
file.write(SectionImageBuilder(flat_section).Svg()) | ||
drawing = svg2rlg("section.svg") | ||
renderPM.drawToFile(drawing, "my_section.png", fmt="PNG") | ||
|
||
|
||
def section_analyse(force=0, moment_yy=0, moment_zz=0): | ||
global section | ||
global design_code | ||
global uls_deformation | ||
global sls_deformation | ||
|
||
print("\nAnalyse section") | ||
solution = IAdSec.Create(design_code).Analyse(section) | ||
strength_results = solution.Strength | ||
serviceability_results = solution.Serviceability | ||
i_load = ILoad.Create(Force(float(force), ForceUnit.Kilonewton), | ||
Moment(float(moment_yy), MomentUnit.KilonewtonMeter), | ||
Moment(float(moment_zz), MomentUnit.KilonewtonMeter)) | ||
uls_deformation = strength_results.Check(i_load).Deformation | ||
sls_deformation = serviceability_results.Check(i_load).Deformation | ||
print("\nULS deformation X " + str(uls_deformation.X.MilliStrain) + | ||
" YY: " + str(uls_deformation.YY.PerMillimeters) + | ||
" zz: " + str(uls_deformation.ZZ.PerMillimeters)) | ||
uls_load_util = strength_results.Check(i_load).LoadUtilisation | ||
print("ULS utilisation: " + str(round(uls_load_util.Percent, 2)) + "%") | ||
|
||
|
||
def section_stress_strain(): | ||
global section | ||
global design_code | ||
global uls_deformation | ||
global sls_deformation | ||
print("\n\nStress - strain at key points of component") | ||
flat_section = IAdSec.Create(design_code).Flatten(section) | ||
corner_points = IPerimeterProfile(flat_section.Profile).SolidPolygon.Points | ||
for points in corner_points: | ||
point = IPoint(points) | ||
y = point.Y.Millimeters | ||
z = point.Z.Millimeters | ||
strain = uls_deformation.StrainAt(point) | ||
stress = flat_section.Material.Strength.StressAt(strain) | ||
print("\nPoint: (" + str(y) + "," + str(z) + ")") | ||
print("ULS Stress: " + str(round(stress.Megapascals, 2)) + | ||
" Strain: " + str(round(strain.MilliStrain, 2))) | ||
strain = sls_deformation.StrainAt(point) | ||
stress = flat_section.Material.Strength.StressAt(strain) | ||
print("SLS Stress: " + str(round(stress.Megapascals, 2)) + | ||
" Strain: " + str(round(strain.MilliStrain, 2))) | ||
|
||
|
||
def rebar_stress_strain(): | ||
global section | ||
global design_code | ||
global uls_deformation | ||
global sls_deformation | ||
print("\n\nULS Stress - strain in rebar") | ||
flat_section = IAdSec.Create(design_code).Flatten(section) | ||
for bar_group in range(1, len(section.ReinforcementGroups)): | ||
rebar_positions = ISingleBars( | ||
flat_section.ReinforcementGroups[bar_group]).Positions | ||
for rebar in rebar_positions: | ||
position = IPoint(rebar) | ||
y = rebar.Y.Millimeters | ||
z = rebar.Z.Millimeters | ||
strain = uls_deformation.StrainAt(position) | ||
stress = ISingleBars(flat_section.ReinforcementGroups[1] | ||
).BarBundle.Material.Strength.StressAt(strain) | ||
print("Point: (" + str(round(y, 1)) + "," + str(round(z, 1)) + | ||
") :" + | ||
" Stress: " + str(round(stress.Megapascals, 1)) + | ||
" Strain: " + str(round(strain.MilliStrain, 2))) | ||
|
||
|
||
if __name__ == "__main__": | ||
print("\nThis AdSec API version is " + IVersion.Api()) | ||
print("____") | ||
|
||
code_ec2_gb() | ||
|
||
new_rectangle_section(700, 500, 30) | ||
add_links(12) | ||
add_top_bars(12, 4) | ||
add_bottom_bars(32, 4) | ||
add_side_bars(16, 150) | ||
section_analyse(0, -450, 0) | ||
section_stress_strain() | ||
|
||
print("____") | ||
|
||
new_circle_section(450, 75) | ||
add_links(12) | ||
add_perimeter_bars(20, 8) | ||
section_image() | ||
section_analyse(-250, 100, 0) | ||
rebar_stress_strain() |
Add this suggestion to a batch that can be applied as a single commit.
This suggestion is invalid because no changes were made to the code.
Suggestions cannot be applied while the pull request is closed.
Suggestions cannot be applied while viewing a subset of changes.
Only one suggestion per line can be applied in a batch.
Add this suggestion to a batch that can be applied as a single commit.
Applying suggestions on deleted lines is not supported.
You must change the existing code in this line in order to create a valid suggestion.
Outdated suggestions cannot be applied.
This suggestion has been applied or marked resolved.
Suggestions cannot be applied from pending reviews.
Suggestions cannot be applied on multi-line comments.
Suggestions cannot be applied while the pull request is queued to merge.
Suggestion cannot be applied right now. Please check back later.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
I had expected links would not be added to the rectangular section, since
global section
was not specified within add_links(), but post line 221, section did have links (I am confused!)My understanding is that any operation on 'section' inside a function would be a local change within that function and shouldn't be reflected on the global section. Ideally I would need to declare global section within add_links() in order for the change to be operated on the global variable. But this isn't what has happened in this script. Same applies to all the rebar types added. Am I missing something?
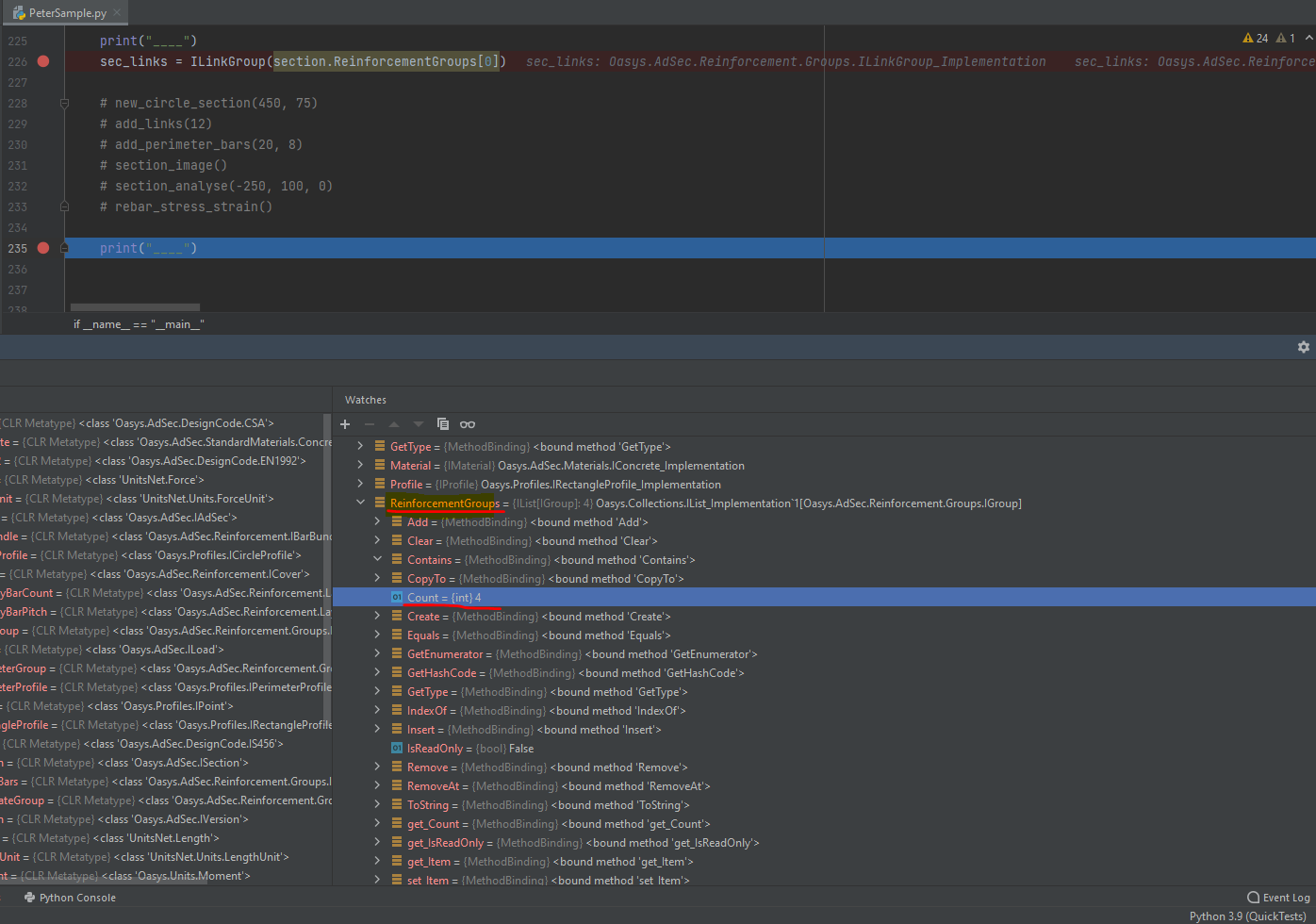
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
@VaradaNambiar I do not understand either why the functions are working without the global definition of the section. My suspicion is that the API is not respecting the local function restrictions in some way, which could be a concern.
@tim-lyon-arup @daviddekoning @nathanrobertsarup do you have any thoughts on this?
Just to be on the safe side I have added "global section" into each rebar function
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
global variables are always accessible in lower scopes in Python. The
global
keyword means that changes in the local scope will be reflected back up to the global scope.If you just want to use a global variable in a lower scope, you do not need to explicitly declare them as global
@PeterDebneyOasys @VaradaNambiar
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
@daviddekoning in this instance, using the section in function changed the global instance, despite the function not declaring it as global, hence the confusion. So the following code does add rebar to the section. Is that as expected?
def add_links(diameter):
global bar_material
bar_dia = Length(float(diameter), LengthUnit.Millimeter)
rebar = ILinkGroup.Create(IBarBundle.Create(bar_material, bar_dia))
section.ReinforcementGroups.Add(rebar)
@VaradaNambiar
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
hi @PeterDebneyOasys @VaradaNambiar , my apologies, I lost track of my github mentions. Did you manage to sort out this issue?