diff --git a/README.md b/README.md
index 0a0889a..3e24e38 100755
--- a/README.md
+++ b/README.md
@@ -94,6 +94,9 @@ The document is being written, please refer to the example source code.
## AlbumView
 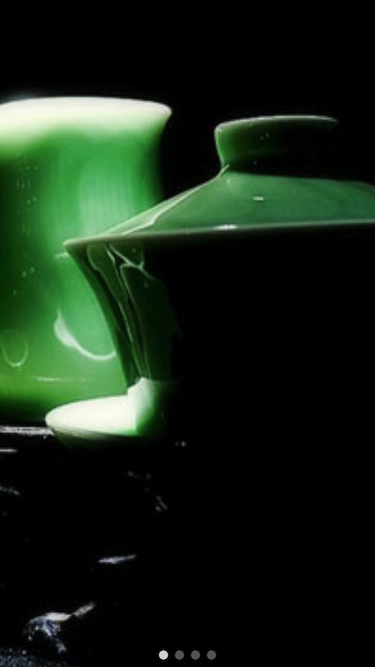
+## Wheel
+
+
## Overlay
 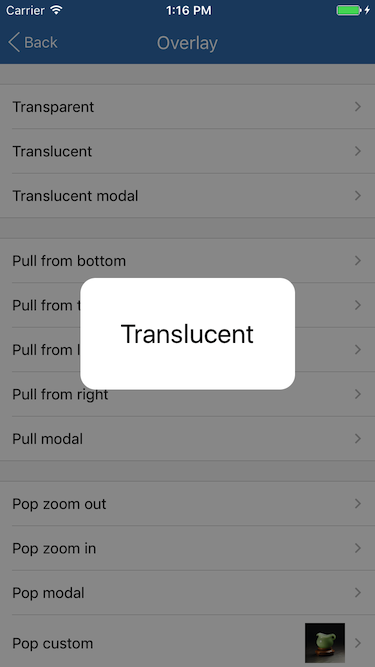
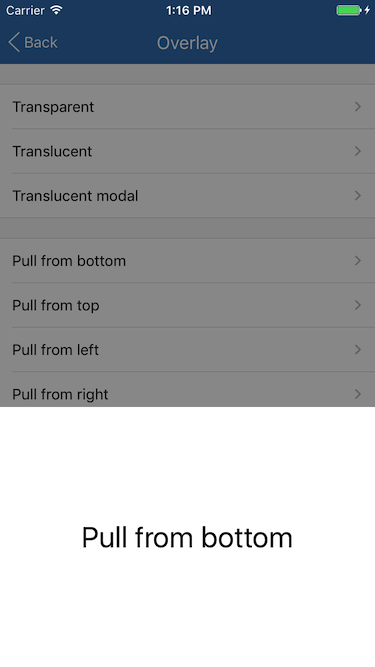 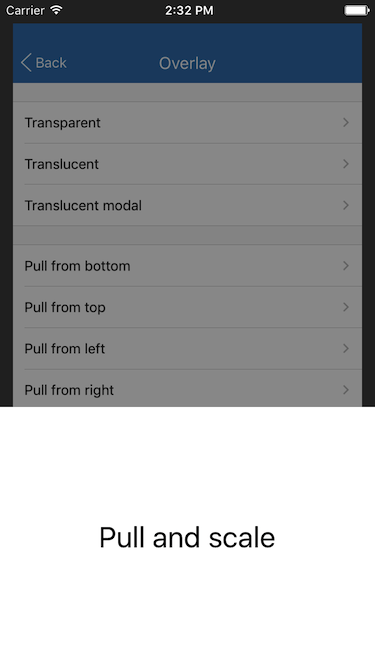
diff --git a/components/ListRow/ListRow.js b/components/ListRow/ListRow.js
index 8b70e58..535875a 100644
--- a/components/ListRow/ListRow.js
+++ b/components/ListRow/ListRow.js
@@ -86,7 +86,8 @@ export default class ListRow extends Component {
//title
if (titlePlace === 'none') {
title = null;
- } if (typeof title === 'string' || typeof title === 'number') {
+ }
+ if (typeof title === 'string' || typeof title === 'number') {
let textStyle = (!detail && titlePlace === 'left') ? {flexGrow: 1, flexShrink: 1} : null;
title =
}
diff --git a/components/Wheel/Wheel.js b/components/Wheel/Wheel.js
new file mode 100644
index 0000000..4fd120b
--- /dev/null
+++ b/components/Wheel/Wheel.js
@@ -0,0 +1,252 @@
+// Wheel.js
+//问题2:不支持受控,对于月份-日期不合法时有问题,如3月31日换到2月
+
+'use strict';
+
+import React, {Component} from "react";
+import PropTypes from 'prop-types';
+import {StyleSheet, View, Text, Animated, PanResponder} from 'react-native';
+
+import Theme from 'teaset/themes/Theme';
+import WheelItem from './WheelItem';
+
+export default class Wheel extends Component {
+
+ static propTypes = {
+ ...View.propTypes,
+ items: PropTypes.arrayOf(PropTypes.oneOfType([PropTypes.element, PropTypes.string, PropTypes.number])).isRequired,
+ itemStyle: Text.propTypes.style,
+ holeStyle: View.propTypes.style, //height is required
+ maskStyle: View.propTypes.style,
+ index: PropTypes.number,
+ defaultIndex: PropTypes.number,
+ onChange: PropTypes.func, //(index)
+ };
+
+ static defaultProps = {
+ ...View.defaultProps,
+ pointerEvents: 'box-only',
+ defaultIndex: 0,
+ };
+
+ static Item = WheelItem;
+ static preRenderCount = 10;
+
+ constructor(props) {
+ super(props);
+ this.createPanResponder();
+ this.prevTouches = [];
+ this.index = props.index || props.index === 0 ? props.index : props.defaultIndex;
+ this.lastRenderIndex = this.index;
+ this.height = 0;
+ this.holeHeight = 0;
+ this.hiddenOffset = 0;
+ this.currentPosition = new Animated.Value(0);
+ this.targetPositionValue = null;
+ }
+
+ componentWillMount() {
+ if (!this.positionListenerId) {
+ this.positionListenerId = this.currentPosition.addListener(e => this.handlePositionChange(e.value));
+ }
+ }
+
+ componentWillUnmount() {
+ if (this.positionListenerId) {
+ this.currentPosition.removeListener(this.positionListenerId);
+ this.positionListenerId = null;
+ }
+ }
+
+ componentWillReceiveProps(nextProps) {
+ if (nextProps.index || nextProps.index === 0) {
+ this.index = nextProps.index;
+ this.currentPosition.setValue(nextProps.index * this.holeHeight);
+ }
+ }
+
+ createPanResponder() {
+ this.panResponder = PanResponder.create({
+ onStartShouldSetPanResponder: (e, gestureState) => true,
+ onStartShouldSetPanResponderCapture: (e, gestureState) => false,
+ onMoveShouldSetPanResponder: (e, gestureState) => true,
+ onMoveShouldSetPanResponderCapture: (e, gestureState) => false,
+ onPanResponderGrant: (e, gestureState) => this.onPanResponderGrant(e, gestureState),
+ onPanResponderMove: (e, gestureState) => this.onPanResponderMove(e, gestureState),
+ onPanResponderTerminationRequest: (e, gestureState) => true,
+ onPanResponderRelease: (e, gestureState) => this.onPanResponderRelease(e, gestureState),
+ onPanResponderTerminate: (e, gestureState) => null,
+ onShouldBlockNativeResponder: (e, gestureState) => true,
+ });
+ }
+
+ onPanResponderGrant(e, gestureState) {
+ this.currentPosition.stopAnimation();
+ this.prevTouches = e.nativeEvent.touches;
+ this.speed = 0;
+ }
+
+ onPanResponderMove(e, gestureState) {
+ let {touches} = e.nativeEvent;
+ let prevTouches = this.prevTouches;
+ this.prevTouches = touches;
+
+ if (touches.length != 1 || touches[0].identifier != prevTouches[0].identifier) {
+ return;
+ }
+
+ let dy = touches[0].pageY - prevTouches[0].pageY;
+ let pos = this.currentPosition._value - dy;
+ this.currentPosition.setValue(pos);
+
+ let t = touches[0].timestamp - prevTouches[0].timestamp;
+ if (t) this.speed = dy / t;
+ }
+
+ onPanResponderRelease(e, gestureState) {
+ this.prevTouches = [];
+ if (Math.abs(this.speed) > 0.1) this.handleSwipeScroll();
+ else this.handleStopScroll();
+ }
+
+ handlePositionChange(value) {
+ let newIndex = Math.round(value / this.holeHeight);
+ if (newIndex != this.index && newIndex >= 0 && newIndex < this.props.items.length) {
+ let moveCount = Math.abs(newIndex - this.lastRenderIndex);
+ this.index = newIndex;
+ if (moveCount > this.constructor.preRenderCount) {
+ this.forceUpdate();
+ }
+ }
+
+ // let the animation stop faster
+ if (this.targetPositionValue != null && Math.abs(this.targetPositionValue - value) <= 2) {
+ this.targetPositionValue = null;
+ this.currentPosition.stopAnimation();
+ }
+ }
+
+ handleSwipeScroll() {
+ let {items} = this.props;
+
+ let inertiaPos = this.currentPosition._value - this.speed * 300;
+ let newIndex = Math.round(inertiaPos / this.holeHeight);
+ if (newIndex < 0) newIndex = 0;
+ else if (newIndex > items.length - 1) newIndex = items.length - 1;
+
+ let toValue = newIndex * this.holeHeight;
+ this.targetPositionValue = toValue;
+ Animated.spring(this.currentPosition, {
+ toValue: toValue,
+ friction: 9,
+ }).start(() => {
+ this.currentPosition.setValue(toValue);
+ this.props.onChange && this.props.onChange(newIndex);
+ });
+ }
+
+ handleStopScroll() {
+ let toValue = this.index * this.holeHeight;
+ this.targetPositionValue = toValue;
+ Animated.spring(this.currentPosition, {
+ toValue: toValue,
+ friction: 9,
+ }).start(() => {
+ this.currentPosition.setValue(toValue);
+ this.props.onChange && this.props.onChange(this.index);
+ });
+ }
+
+ handleLayout(height, holeHeight) {
+ this.height = height;
+ this.holeHeight = holeHeight;
+ if (holeHeight) {
+ let maskHeight = (height - holeHeight) / 2;
+ this.hiddenOffset = Math.ceil(maskHeight / holeHeight) + this.constructor.preRenderCount;
+ }
+ this.forceUpdate(() => this.currentPosition.setValue(this.index * holeHeight));
+ }
+
+ onLayout(e) {
+ this.handleLayout(e.nativeEvent.layout.height, this.holeHeight);
+ this.props.onLayout && this.props.onLayout(e);
+ }
+
+ onHoleLayout(e) {
+ this.handleLayout(this.height, e.nativeEvent.layout.height);
+ }
+
+ buildProps() {
+ let {style, items, itemStyle, holeStyle, maskStyle, ...others} = this.props;
+
+ style = [{
+ backgroundColor: Theme.wheelColor,
+ overflow: 'hidden',
+ }].concat(style);
+ itemStyle = [{
+ backgroundColor: 'rgba(0, 0, 0, 0)',
+ fontSize: Theme.wheelFontSize,
+ color: Theme.wheelTextColor,
+ }].concat(itemStyle);
+ holeStyle = [{
+ backgroundColor: 'rgba(0, 0, 0, 0)',
+ height: Theme.wheelHoleHeight,
+ borderColor: Theme.wheelHoleLineColor,
+ borderTopWidth: Theme.wheelHoleLineWidth,
+ borderBottomWidth: Theme.wheelHoleLineWidth,
+ zIndex: 1,
+ }].concat(holeStyle);
+ maskStyle = [{
+ backgroundColor: Theme.wheelMaskColor,
+ opacity: Theme.wheelMaskOpacity,
+ flex: 1,
+ zIndex: 100,
+ }].concat(maskStyle);
+
+ this.props = {style, items, itemStyle, holeStyle, maskStyle, ...others};
+ }
+
+ renderItem(item, itemIndex) {
+ let {itemStyle} = this.props;
+
+ if (Math.abs(this.index - itemIndex) > this.hiddenOffset) return null;
+
+ if (typeof item === 'string' || typeof item === 'number') {
+ item = {item};
+ }
+
+ return (
+
+ {item}
+
+ );
+ }
+
+ render() {
+ this.buildProps();
+ this.lastRenderIndex = this.index;
+
+ let {items, itemStyle, holeStyle, maskStyle, defaultIndex, onChange, onLayout, ...others} = this.props;
+
+ return (
+ this.onLayout(e)}
+ {...this.panResponder.panHandlers}
+ >
+ {items.map((item, index) => this.renderItem(item, index))}
+
+ this.onHoleLayout(e)} />
+
+
+ )
+ }
+
+}
+
diff --git a/components/Wheel/WheelItem.js b/components/Wheel/WheelItem.js
new file mode 100644
index 0000000..5949702
--- /dev/null
+++ b/components/Wheel/WheelItem.js
@@ -0,0 +1,126 @@
+// WheelItem.js
+
+'use strict';
+
+import React, {Component} from "react";
+import PropTypes from 'prop-types';
+import {StyleSheet, View, Text, Animated} from 'react-native';
+
+import Theme from 'teaset/themes/Theme';
+
+export default class WheelItem extends Component {
+
+ static propTypes = {
+ ...Animated.View.propTypes,
+ index: PropTypes.number.isRequired,
+ itemHeight: PropTypes.number.isRequired,
+ wheelHeight: PropTypes.number.isRequired,
+ currentPosition: PropTypes.any, //instanceOf(Animated)
+ };
+
+ static defaultProps = {
+ ...Animated.View.defaultProps,
+ };
+
+ constructor(props) {
+ super(props);
+ this.lastPosition = null;
+ this.state = {
+ translateY: new Animated.Value(100000),
+ scaleX: new Animated.Value(1),
+ scaleY: new Animated.Value(1),
+ };
+ }
+
+ componentWillMount() {
+ if (!this.positionListenerId) {
+ this.positionListenerId = this.props.currentPosition.addListener(e => {
+ this.handlePositionChange(e.value);
+ });
+ this.handlePositionChange(this.props.currentPosition._value);
+ }
+ }
+
+ componentWillUnmount() {
+ if (this.positionListenerId) {
+ this.props.currentPosition.removeListener(this.positionListenerId);
+ this.positionListenerId = null;
+ }
+ }
+
+ componentWillReceiveProps(nextProps) {
+ let {itemHeight, wheelHeight, index} = this.props;
+ if (nextProps.index != index
+ || nextProps.itemHeight != itemHeight
+ || nextProps.wheelHeight != wheelHeight) {
+ this.handlePositionChange(nextProps.currentPosition._value, nextProps);
+ }
+ }
+
+ calcProjection(diameter, point, width) {
+ if (diameter == 0) return false;
+ let radius = diameter / 2;
+ let circumference = Math.PI * diameter;
+ let quarter = circumference / 4;
+ if (Math.abs(point) > quarter) return false;
+ let alpha = point / circumference * Math.PI * 2;
+
+ let pointProjection = radius * Math.sin(alpha);
+ let distance = radius - radius * Math.sin(Math.PI / 2 - alpha);
+ let eyesDistance = 1000;
+ let widthProjection = width * eyesDistance / (distance + eyesDistance);
+
+ return {point: pointProjection, width: widthProjection};
+ }
+
+ handlePositionChange(value, props = null) {
+ let {itemHeight, wheelHeight, index} = props ? props : this.props;
+
+ if (!itemHeight || !wheelHeight) return;
+ if (this.lastPosition !== null && Math.abs(this.lastPosition - value) < 1) return;
+
+ let itemPosition = itemHeight * index;
+ let halfItemHeight = itemHeight / 2;
+ let top = itemPosition - value - halfItemHeight;
+ let bottom = top + itemHeight;
+ let refWidth = 100;
+ let p1 = this.calcProjection(wheelHeight, top, refWidth);
+ let p2 = this.calcProjection(wheelHeight, bottom, refWidth);
+
+ let ty = 10000, sx = 1, sy = 1;
+ if (p1 && p2) {
+ let y1 = p1.point;
+ let y2 = p2.point;
+ ty = (y1 + y2) / 2;
+ sy = (y2 - y1) / itemHeight;
+ sx = (p1.width + p2.width) / 2 / refWidth;
+ }
+
+ let {translateY, scaleX, scaleY} = this.state;
+ translateY.setValue(ty);
+ scaleX.setValue(sx);
+ scaleY.setValue(sy);
+ this.lastPosition = value;
+ }
+
+ render() {
+ let {style, itemHeight, wheelHeight, index, currentPosition, children, ...others} = this.props;
+ let {translateY, scaleX, scaleY} = this.state;
+ style = [{
+ backgroundColor: 'rgba(0, 0, 0, 0)',
+ position: 'absolute',
+ left: 0,
+ right: 0,
+ top: 0,
+ bottom: 0,
+ justifyContent: 'center',
+ transform: [{scaleX}, {translateY}, {scaleY}],
+ }].concat(style);
+ return (
+
+ {children}
+
+ );
+ }
+
+}
diff --git a/docs/cn/AlbumView.md b/docs/cn/AlbumView.md
index 67d8e35..2d7f27a 100644
--- a/docs/cn/AlbumView.md
+++ b/docs/cn/AlbumView.md
@@ -5,7 +5,7 @@ AlbumView 组件定义一个相册视图, 支持多图左右切换显示,支
| Prop | Type | Default | Note |
|---|---|---|---|
| [View props...](https://facebook.github.io/react-native/docs/view.html) | | | AlbumView 组件继承 View 组件的全部属性。
-| images | array | | 相册图片数组,必填,数组元素为 Image.source 。
+| images | array | | 相册图片数组,必填,数组元素为 Image.source 或 React Native 组件。
| thumbs | array | | 相册缩略图数组,可空,数组元素为 Image.source 。
| defaultIndex | number | 0 | 默认显示图片索引。
| index | number | | 显示图片索引,设置此属性需要监听 onChange 事件并自行维护状态。
diff --git a/docs/cn/README.md b/docs/cn/README.md
index e931517..803b5b4 100644
--- a/docs/cn/README.md
+++ b/docs/cn/README.md
@@ -108,6 +108,8 @@ react-native run-android
[`` 相册视图](./AlbumView.md)
+[`` 滚轮](./Wheel.md)
+
## 浮层
[`Overlay{}` 浮层](./Overlay.md)
diff --git a/docs/cn/Select.md b/docs/cn/Select.md
index b4979b8..0f94164 100644
--- a/docs/cn/Select.md
+++ b/docs/cn/Select.md
@@ -10,7 +10,7 @@ Select 组件定义一个选择框。
| valueStyle | 同Text.style | | 值显示样式。
| items | array | | 可选择列表数组, 数组元素可以是任何类型。
| getItemValue | func | | 取 items 数组元素的 value 值, 传入参数为(item, index), item = items[index], 默认直接使用 item
-| getItemText | func | | 取 items 数组元素的显示文本, 传入参数为(item, index), item = items[index], 默认直接使用 item
+| getItemText | func | | 取 items 数组元素的显示文本或 React Native 组件, 传入参数为(item, index), item = items[index], 默认直接使用 item
| pickerType | string | 'auto' | 选择器类型。
- auto: 自动选择, 当设备为 Pad(宽和高均大于768)时使用 PopoverPicker, 否则使用 PullPicker
- pull: PullPicker
- popover: PopoverPicker
| pickerTitle | string | | PullPicker 选择器标题。
| editable | bool | true | 是否可编辑。
diff --git a/docs/cn/Wheel.md b/docs/cn/Wheel.md
new file mode 100644
index 0000000..8d6c004
--- /dev/null
+++ b/docs/cn/Wheel.md
@@ -0,0 +1,43 @@
+# `` 滚轮
+Wheel 组件定义一个滚轮, 可用于滚轮式选择器,同时支持 Android 和 iOS。
+
+## Props
+| Prop | Type | Default | Note |
+|---|---|---|---|
+| [View props...](https://facebook.github.io/react-native/docs/view.html) | | | Wheel 组件继承 View 组件的全部属性。
+| items | array | | 可选项列表,数组元素可以是字符串、数字或 React Native 组件。
+| itemStyle | 同Text.style | | 选项样式,当 items 数组元素是 React Native 组件时无效。
+| holeStyle | 同View.style | | 当前项窗口样式,需指定 height 属性。
+| maskStyle | 同View.style | | 当前项上下蒙版样式。
+| index | number | | 当前项索引值,设置此属性需要监听 onChange 事件并自行维护状态。
+| defaultIndex | number | | 默认当前项索引值,仅在组件创建时使用一次,如不想设置 index 并维护状态,可在此属性传入初始项索引值。
+
+## Events
+| Event Name | Returns | Notes |
+|---|---|---|
+| [View events...](https://facebook.github.io/react-native/docs/view.html) | | Wheel 组件继承 View 组件的全部事件。
+| onChange | index | 改变当前项完成后调用, index 为改变后当前项索引值。
+
+
+
+## Example
+简单用法
+```
+
+```
+
+## Screenshots
+
diff --git a/example/views/Home.js b/example/views/Home.js
index 3246623..1e8ac59 100644
--- a/example/views/Home.js
+++ b/example/views/Home.js
@@ -25,6 +25,7 @@ import SegmentedViewExample from './SegmentedViewExample';
import TabViewExample from './TabViewExample';
import TransformViewExample from './TransformViewExample';
import AlbumViewExample from './AlbumViewExample';
+import WheelExample from './WheelExample';
import OverlayExample from './OverlayExample';
import ToastExample from './ToastExample';
import ActionSheetExample from './ActionSheetExample';
@@ -65,6 +66,7 @@ export default class Home extends NavigationPage {
this.navigator.push({view: })} />
this.navigator.push({view: })} />
this.navigator.push({view: })} />
+ this.navigator.push({view: })} />
this.navigator.push({view: })} />
this.navigator.push({view: })} />
this.navigator.push({view: })} />
diff --git a/example/views/WheelExample.js b/example/views/WheelExample.js
new file mode 100644
index 0000000..d0c2b24
--- /dev/null
+++ b/example/views/WheelExample.js
@@ -0,0 +1,88 @@
+// WheelExample.js
+
+'use strict';
+
+import React, {Component} from 'react';
+import {View} from 'react-native';
+
+import {NavigationPage, Theme, Wheel} from 'teaset';
+
+export default class WheelExample extends NavigationPage {
+
+ static defaultProps = {
+ ...NavigationPage.defaultProps,
+ title: 'Wheel',
+ showBackButton: true,
+ };
+
+ constructor(props) {
+ super(props);
+ this.years = [];
+ for (let i = 1970; i <= 2100; ++i) this.years.push(i);
+ this.months = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12];
+ this.daysCount = [
+ [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31],
+ [31, 29, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31],
+ ];
+ Object.assign(this.state, {
+ date: new Date(),
+ });
+ }
+
+ isLeapYear(year) {
+ return (year % 4 == 0) && (year % 100 != 0 || year % 400 == 0);
+ }
+
+ onDateChange(year, month, day) {
+ let {date} = this.state;
+ date.setFullYear(year);
+
+ let daysCount = this.daysCount[this.isLeapYear(year) ? 1 : 0][month];
+ if (day > daysCount) {
+ day = daysCount;
+ date.setDate(day);
+ date.setMonth(month);
+ } else {
+ date.setMonth(month);
+ date.setDate(day);
+ }
+ this.setState({date});
+ }
+
+ renderPage() {
+ let {date} = this.state;
+ let year = date.getFullYear(), month = date.getMonth(), day = date.getDate();
+ let daysCount = this.daysCount[this.isLeapYear(year) ? 1 : 0][month];
+ let days = [];
+ for (let i = 1; i <= daysCount; ++i) days.push(i);
+ return (
+
+
+
+ this.onDateChange(this.years[index], month, day)}
+ />
+ this.onDateChange(year, this.months[index] - 1, day)}
+ />
+ this.onDateChange(year, month, days[index])}
+ />
+
+
+ );
+ }
+
+}
diff --git a/index.js b/index.js
index b3556f3..f870a37 100644
--- a/index.js
+++ b/index.js
@@ -22,6 +22,7 @@ import SegmentedView from './components/SegmentedView/SegmentedView';
import TabView from './components/TabView/TabView';
import TransformView from './components/TransformView/TransformView';
import AlbumView from './components/AlbumView/AlbumView';
+import Wheel from './components/Wheel/Wheel';
import Overlay from './components/Overlay/Overlay';
import Toast from './components/Toast/Toast';
@@ -61,6 +62,7 @@ var Teaset = {
TabView,
TransformView,
AlbumView,
+ Wheel,
Overlay,
Toast,
diff --git a/package.json b/package.json
index bca1f2c..f047652 100644
--- a/package.json
+++ b/package.json
@@ -1,6 +1,6 @@
{
"name": "teaset",
- "version": "0.3.4",
+ "version": "0.4.0",
"description": "A UI library for react native.",
"main": "index.js",
"scripts": {
diff --git a/screenshots/14b-Wheel.png b/screenshots/14b-Wheel.png
new file mode 100644
index 0000000..28c8409
Binary files /dev/null and b/screenshots/14b-Wheel.png differ
diff --git a/themes/ThemeBlack.js b/themes/ThemeBlack.js
index 075a6c4..46bdcb6 100644
--- a/themes/ThemeBlack.js
+++ b/themes/ThemeBlack.js
@@ -303,6 +303,16 @@ export default {
carouselDotColor: 'rgba(255, 255, 255, 0.4)',
carouselActiveDotColor: 'rgba(255, 255, 255, 0.85)',
+ //Wheel
+ wheelColor: defaultColor,
+ wheelFontSize: 14,
+ wheelTextColor: defaultTextColor,
+ wheelHoleHeight: 28,
+ wheelHoleLineWidth: pixelSize,
+ wheelHoleLineColor: '#555',
+ wheelMaskColor: defaultColor,
+ wheelMaskOpacity: 0.4,
+
//Overlay
overlayOpacity: 0.4,
overlayRootScale: 0.93,
diff --git a/themes/ThemeDefault.js b/themes/ThemeDefault.js
index 40f101d..556db21 100644
--- a/themes/ThemeDefault.js
+++ b/themes/ThemeDefault.js
@@ -303,6 +303,16 @@ export default {
carouselDotColor: 'rgba(255, 255, 255, 0.4)',
carouselActiveDotColor: 'rgba(255, 255, 255, 0.85)',
+ //Wheel
+ wheelColor: defaultColor,
+ wheelFontSize: 14,
+ wheelTextColor: defaultTextColor,
+ wheelHoleHeight: 28,
+ wheelHoleLineWidth: pixelSize,
+ wheelHoleLineColor: '#ccc',
+ wheelMaskColor: defaultColor,
+ wheelMaskOpacity: 0.4,
+
//Overlay
overlayOpacity: 0.4,
overlayRootScale: 0.93,
diff --git a/themes/ThemeViolet.js b/themes/ThemeViolet.js
index 11b66ab..d306fc5 100644
--- a/themes/ThemeViolet.js
+++ b/themes/ThemeViolet.js
@@ -303,6 +303,16 @@ export default {
carouselDotColor: 'rgba(255, 255, 255, 0.4)',
carouselActiveDotColor: 'rgba(255, 255, 255, 0.85)',
+ //Wheel
+ wheelColor: defaultColor,
+ wheelFontSize: 14,
+ wheelTextColor: defaultTextColor,
+ wheelHoleHeight: 28,
+ wheelHoleLineWidth: pixelSize,
+ wheelHoleLineColor: '#494d5e',
+ wheelMaskColor: defaultColor,
+ wheelMaskOpacity: 0.4,
+
//Overlay
overlayOpacity: 0.4,
overlayRootScale: 0.93,