|
1 |
| -[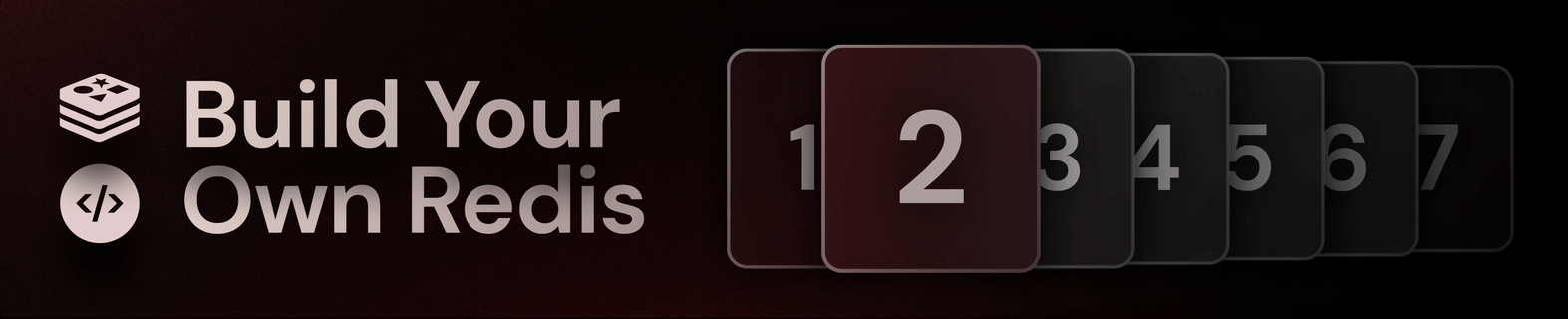](https://app.codecrafters.io/users/codecrafters-bot?r=2qF) |
| 1 | +# Build Your Own Redis |
2 | 2 |
|
3 |
| -This is a starting point for Rust solutions to the |
4 |
| -["Build Your Own Redis" Challenge](https://codecrafters.io/challenges/redis). |
| 3 | +To build a toy Redis-Server clone that's capable of handling |
| 4 | +basic commands like `PING`, `SET` and `GET`. Also implement the event loops, the Redis protocol and more. |
5 | 5 |
|
6 |
| -In this challenge, you'll build a toy Redis clone that's capable of handling |
7 |
| -basic commands like `PING`, `SET` and `GET`. Along the way we'll learn about |
8 |
| -event loops, the Redis protocol and more. |
9 |
| - |
10 |
| -**Note**: If you're viewing this repo on GitHub, head over to |
11 |
| -[codecrafters.io](https://codecrafters.io) to try the challenge. |
12 |
| - |
13 |
| -# Passing the first stage |
| 6 | +## Prerequisites |
| 7 | +install `redis-cli` first (an implementation of redis client for test purpose) |
| 8 | +```sh |
| 9 | +cargo install mini-redis |
| 10 | +``` |
14 | 11 |
|
15 |
| -The entry point for your Redis implementation is in `src/main.rs`. Study and |
16 |
| -uncomment the relevant code, and push your changes to pass the first stage: |
17 | 12 |
|
| 13 | +## Supported Commands |
18 | 14 | ```sh
|
19 |
| -git commit -am "pass 1st stage" # any msg |
20 |
| -git push origin master |
| 15 | +redis-cli PING |
21 | 16 | ```
|
22 | 17 |
|
23 |
| -That's all! |
24 |
| - |
25 |
| -# Stage 2 & beyond |
| 18 | +```sh |
| 19 | +redis-cli ECHO hey |
| 20 | +``` |
26 | 21 |
|
27 |
| -Note: This section is for stages 2 and beyond. |
| 22 | +```sh |
| 23 | +redis-cli SET foo bar |
| 24 | +``` |
28 | 25 |
|
29 |
| -1. Ensure you have `cargo (1.54)` installed locally |
30 |
| -1. Run `./your_program.sh` to run your Redis server, which is implemented in |
31 |
| - `src/main.rs`. This command compiles your Rust project, so it might be slow |
32 |
| - the first time you run it. Subsequent runs will be fast. |
33 |
| -1. Commit your changes and run `git push origin master` to submit your solution |
34 |
| - to CodeCrafters. Test output will be streamed to your terminal. |
| 26 | +```sh |
| 27 | +redis-cli GET foo |
| 28 | +``` |
0 commit comments