Commit 12161af
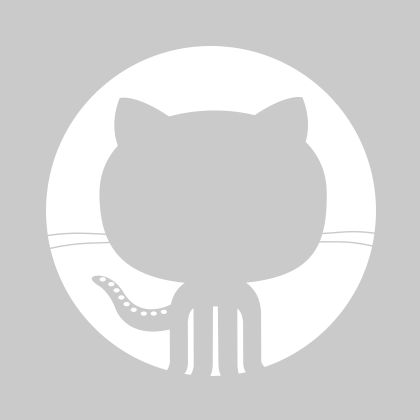
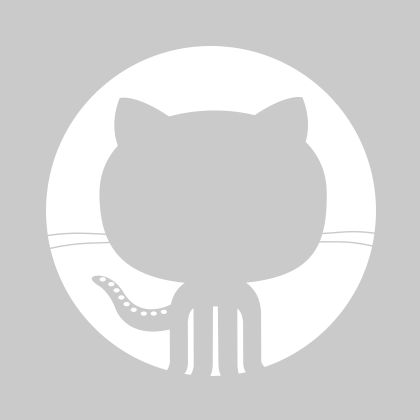
Ville Koskela
Ville Koskela
1 parent 159e268 commit 12161af
File tree
5 files changed
+83
-14
lines changed- src
- main/java/com/fasterxml/jackson/databind
- deser
- type
- test/java/com/fasterxml/jackson/databind
- deser/builder
- type
5 files changed
+83
-14
lines changedOriginal file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
198 | 198 |
| |
199 | 199 |
| |
200 | 200 |
| |
| 201 | + | |
| 202 | + | |
| 203 | + | |
| 204 | + | |
| 205 | + | |
| 206 | + | |
| 207 | + | |
| 208 | + | |
| 209 | + | |
| 210 | + | |
| 211 | + | |
| 212 | + | |
201 | 213 |
| |
202 | 214 |
| |
203 | 215 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
138 | 138 |
| |
139 | 139 |
| |
140 | 140 |
| |
141 |
| - | |
142 |
| - | |
143 |
| - | |
| 141 | + | |
| 142 | + | |
| 143 | + | |
| 144 | + | |
144 | 145 |
| |
145 | 146 |
| |
146 |
| - | |
| 147 | + | |
| 148 | + | |
| 149 | + | |
| 150 | + | |
| 151 | + | |
| 152 | + | |
147 | 153 |
| |
148 | 154 |
| |
149 | 155 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
894 | 894 |
| |
895 | 895 |
| |
896 | 896 |
| |
897 |
| - | |
| 897 | + | |
| 898 | + | |
| 899 | + | |
| 900 | + | |
| 901 | + | |
| 902 | + | |
| 903 | + | |
| 904 | + | |
| 905 | + | |
| 906 | + | |
| 907 | + | |
| 908 | + | |
| 909 | + | |
| 910 | + | |
| 911 | + | |
| 912 | + | |
| 913 | + | |
| 914 | + | |
| 915 | + | |
| 916 | + | |
| 917 | + | |
| 918 | + | |
| 919 | + | |
898 | 920 |
| |
899 | 921 |
| |
900 | 922 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 |
| - | |
| 1 | + | |
2 | 2 |
| |
3 |
| - | |
4 |
| - | |
5 |
| - | |
| 3 | + | |
| 4 | + | |
6 | 5 |
| |
7 |
| - | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
8 | 9 |
| |
| 10 | + | |
| 11 | + | |
9 | 12 |
| |
10 |
| - | |
| 13 | + | |
11 | 14 |
| |
12 | 15 |
| |
13 | 16 |
| |
| |||
77 | 80 |
| |
78 | 81 |
| |
79 | 82 |
| |
80 |
| - | |
| 83 | + | |
| 84 | + | |
| 85 | + | |
| 86 | + | |
| 87 | + | |
| 88 | + | |
| 89 | + | |
| 90 | + | |
| 91 | + | |
| 92 | + | |
| 93 | + | |
| 94 | + | |
| 95 | + | |
81 | 96 |
| |
82 | 97 |
| |
83 | 98 |
| |
84 | 99 |
| |
85 | 100 |
| |
86 | 101 |
| |
87 | 102 |
| |
88 |
| - | |
| 103 | + | |
89 | 104 |
| |
90 | 105 |
| |
91 | 106 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
175 | 175 |
| |
176 | 176 |
| |
177 | 177 |
| |
178 |
| - | |
| 178 | + | |
| 179 | + | |
| 180 | + | |
| 181 | + | |
| 182 | + | |
| 183 | + | |
| 184 | + | |
| 185 | + | |
179 | 186 |
| |
180 | 187 |
| |
181 | 188 |
| |
182 | 189 |
| |
183 | 190 |
| |
184 | 191 |
| |
| 192 | + | |
| 193 | + | |
| 194 | + | |
| 195 | + | |
| 196 | + | |
| 197 | + | |
| 198 | + | |
185 | 199 |
| |
186 | 200 |
| |
187 | 201 |
| |
|
0 commit comments